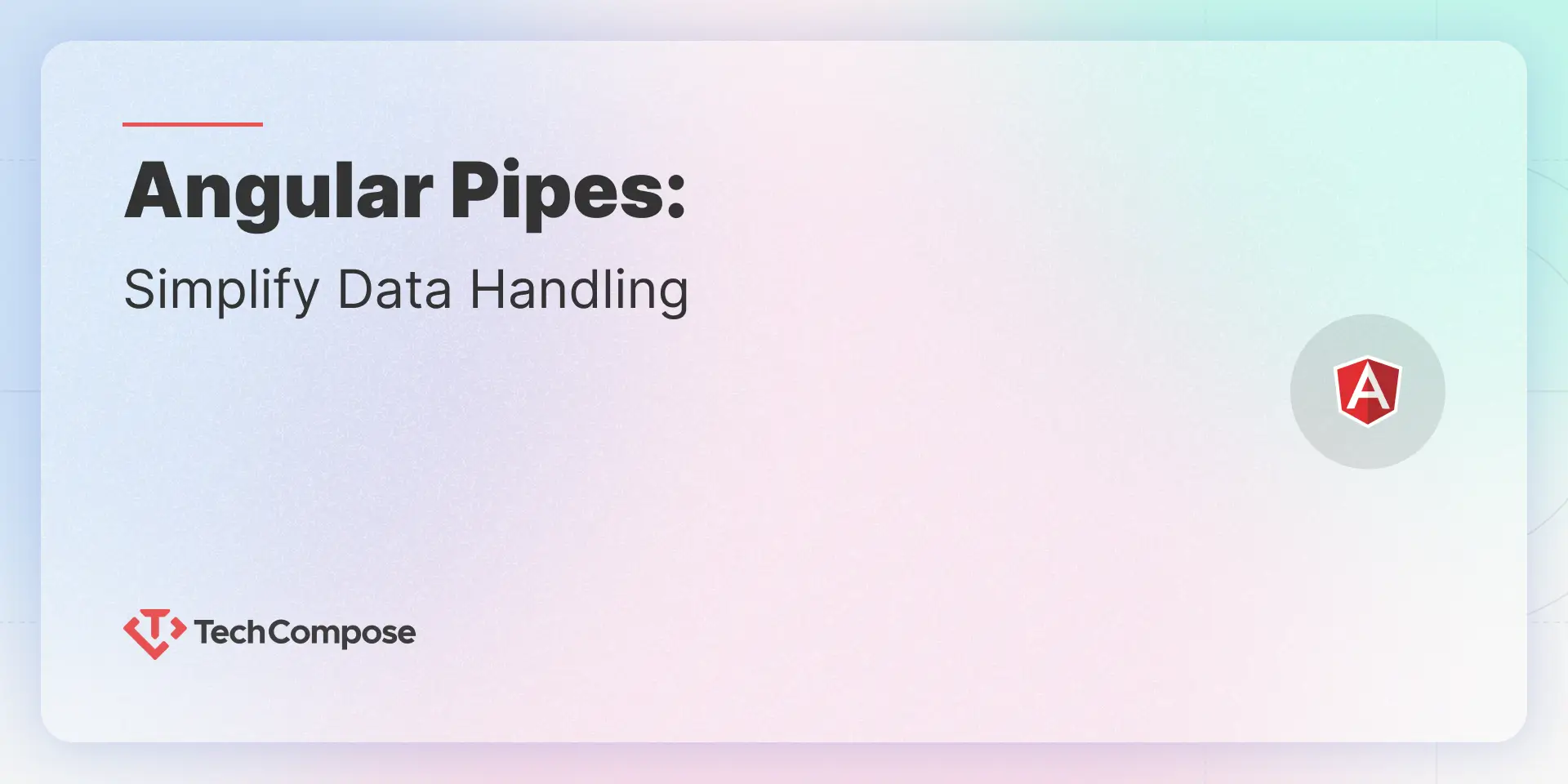
Table of Contents
Let’s discover the power of pipes together! ✨
Introduction to Angular Pipes with its types
Why use Angular pipes?
Readability and Clean Template
It allows data to be transformed directly in the HTML template using built-in or custom pipes. This enhances readability, keeps the HTML code clean, and ensures that users see properly formatted data.
Code Reusability
We can also create custom pipes, allowing us to transform or format data across multiple components and templates with a single pipe, thereby reducing code duplication.
Performance optimization
Angular pipes are designed to enhance performance, as pure pipes use the change detection mechanism to transform data efficiently into a readable view.
How to use Pipes in Angular Template
To use a pipe in a template expression, insert the pipe symbol (|).
Let’s investigate the types of Angular pipes, we have 2 types of angular pipes:
- Pure Pipes
- Impure Pipes
Pure Pipes
Pure pipe is a type of pipe that is designed to be highly efficient. It processes data only when there’s a pure change in the input. A pure change occurs when the Angular change detection system identifies a change in either a primitive input value (such as a string, number, Boolean, or symbol) or an object reference (like a Date, Array, Function, or Object).
Now we will see the few pure in-build pipes that Angular itself provides.
1. Date Pipe
The built-in Date Pipe in Angular formats date objects or date strings into user-friendly date and time representations in Angular templates. It provides a way to display dates in different formats, such as short dates, long dates, time, and more, making it easy to present date information to users in a readable manner.
In the Ts file, I have stored the today’s date in one variable
public todaysDate = new Date();
So directly if we present this date to the end-user then it will display with India standard time, and it does not look like a properly formatted date
Mon Nov 06 2023 14:55:31 GMT+0530 (India Standard Time)
So to display the properly formatted date we will now use the date pipe function
{{ todaysDate | date }}
Output: - Nov 6, 2023
2. Decimal Pipe
Decimal Pipe in Angular is used to format numeric values with decimal places. It enables you to control the number of decimal places, as well as the use of thousands of separators, making numbers easier to read and understand in Angular templates.
Here we have one variable with a floating value
public num = 54.6786594;
The value of this variable is 54.6786594. So, at decimal places, we have 7 numbers. To format decimal places with 2-3 numbers we can use decimal pipe like this
The number with formatted decimal places
{{ num | number }}
Output: - 54.678
By default, decimal Pipe format up to 3 numbers. And to customize that number we need to use parameters that we will look at later in this blog.
3. Currency Pipe
Currency Pipe in Angular is used to format numeric values as currency, making it easy to display monetary amounts in a user-friendly way in Angular templates. It allows you to specify the currency code, symbol, and locale to tailor the currency presentation to your requirements.
public amount: number = 10;
{{ amount | currency }}
Output:- $10.00
In this example, the Currency Pipe is used to format the amount value as currency using the specified currency code. It will display the number with the currency symbol and code, such as “$10.00”.
By default, currency pipe will display a dollar sign, for other country currency codes later we will look into how to use parameters.
4. UpperCase Pipe
The UppercasePipe is used to transform an entire string to uppercase.
public text: string = 'hello angular!';
{{ text | uppercase }}
Output:- HELLO ANGULAR!
5. LowerCase Pipe
The LowerCase Pipe is used to transform an entire string to lowercase.
public text: string = 'Hello Angular!';
{{ text | uppercase }}
Output:- hello angular!
6. TitleCase Pipe
The TitleCasePipe is used to capitalize the first letter of each word, while the rest of the letters in each word are converted to lowercase.
public text: string = 'hello angular
{{ text | uppercase }}
Output:- Hello Angular!
7. Percent Pipe
The Percent pipe is used to transform number into percentage string
public percentageValue: number = 0.779
{{ percentageValue | percent }}
Output:- 78%
Impure Pipes
Impure pipes, on the other hand, are less efficient in terms of performance. Impure pipes are typically used when the output is influenced by factors beyond the direct changes in input data, such as timers, asynchronous events, adding an element to the existing array or object, and changes within the object value.
Now we will see the few impure in-build pipes that Angular itself provides.
1. Async Pipe
The Angular inbuilt async pipe is a feature in the Angular framework that simplifies the process of working with asynchronous data and observables in templates. It allows you to directly bind to asynchronous data sources, such as Promises or Observables, in your HTML templates without having to manually subscribe to them in your component code.
Create one service with the name “my-service”. In that define one behaviour subject of type string and write that function.
export class MyServiceService {
/** status subject */
public statusSubject: BehaviorSubject = new BehaviorSubject('');
constructor() { }
/** Set status */
public setStatus(status: string): void {
this.statusSubject.next(status);
}
}
Now set the status from the TS file using this behavior subject
this.myService.setStatus('Hello Everyone!')
So, if we want to display this status to the user in HTML as per the general process then first, we create a variable of type string then we subscribe this behaviour subject and whatever the response we get from this, we store it to that variable and then we display it in HTML.
This is something a lengthy process😮💨. So instead, we need to inject this service with the public in the TS file to access outside the TS file and then through the Async pipe, we can directly use this service into the template
constructor(
public myService: MyServiceService
) {
/** no data */
}
{{ (myService.statusSubject | async) }}
Output:- Hello Everyone!
2. JSON Pipe
JSON Pipe is used to transform an Object into JSON representation. This is useful for debugging
public book: Object = {
name: 'Treasure Island'
author: 'By Robert Louis Stevenson'
};
{{ book | json }}
Output:- { "name": "Treasure Island", "author": "By Robert Louis Stevenson" }
Let’s quickly go through the difference between pure and impure pipe:
Pure pipe | Impure pipe | |
Works When | Only when the input value or reference changes (e.g., a new primitive value or object reference). | Runs on every change detection cycle, even if the input value or reference hasn’t changed. |
Data Detection | Does not detect changes within objects or arrays because it relies on reference comparison. | Detects changes within objects or arrays (e.g., adding, removing, or modifying elements/properties). |
Performance | Optimized for performance by avoiding unnecessary recalculations. | Reduces performance as it runs frequently. |
Default Behaviour | The default behaviour of any Angular pipe is pure. | Must explicitly set pure: false in the @Pipe decorator. |
Use Case | Ideal for formatting static or immutable data, like dates, currencies, or numbers. | Suitable for dynamic data, like filtering or formatting arrays and object values that may change frequently. |
Key Difference
Pure Pipes: Efficient, rely on input value/reference changes, great for static data.
Impure Pipes: Less efficient, run frequently, handle dynamic data changes (e.g.,
within arrays/objects).
Parameterized Pipes
A parameterized pipe is a custom data transformation function that accepts one or more parameters in your template to modify the output based on the provided arguments.
We can provide a parameter to the pipe followed by a colon (:) A pipe symbol after that specific pipe name then a colon and then a parameter according to the pipe
Let’s check the Date Pipe with the parameters
By default, date pipe provides the format like this “Nov 6, 2023”, but if we want to present the date to the end user in a different format for example only want to display short-Date, then we can do like this
public todaysDate = new Date();
{{ todaysDate | date: 'M/d/yy' }}
Output: 11/09/23
So, like this Date Pipe, we can also modify all other pipe default formats through parameters. Here are just a few pipe examples links in which we can use parameters.
• Decimal Pipe
By default, decimal pipe provides format like this “3.142”, but if we want to manipulate decimal pipe with some parameters then we can do like this
Syntax of decimal pipe parameters
Syntax: “minIntegerDigits.minFractionDigits-maxFractionDigits”
public value: number = '19.6746739'
{{ value | number: '1.1-2' }}
Output: 19.68
• Currency Pipe
By default, the Angular CurrencyPipe uses the en-US locale, which displays the US Dollar ($) sign. If you want to use a different country code or currency symbol, you can explicitly specify it in the pipe’s parameters.
{{ 1234.56 | currency }}
{{ 1234.56 | currency: 'EUR' }}
{{ 1234.56 | currency: 'INR' }}
Chaining Pipes: Combining Transformations
“Chaining pipes” refers to the practice of applying multiple Angular pipes consecutively to transform and format data in a template
For example, you might have a data-binding expression in your template like this:
public todaysDate = new Date();
{{ todaysDate | date: 'EEEE, MMMM d, y' | uppercase }}
Output: THURSDAY, NOVEMBER 9, 2023
Here in this example, we have used the date pipe with parameters along with we have used uppercase pipe to transform the string in capitalize.
How to use angular pipes in the TS file
Till now we have seen examples of using the pipes in the template. So now let’s check how we can use pipe in the TS file
first, we must inject this Title case pipe into the constructor:
constructor(
private titleCasePipe: TitleCasePipe) {
/* no data */
});
}
Then after injecting, we can use it in any function call. Here we will see an example of using Title Case pipe on key up event
HTML File
TS File
public capitalizeFirstChar(): void {
this.form.get('name').patchValue(this.titleCasePipe.transform(this.form.get(
'name').value)))
}
Creating Custom Pipes
A custom pipe in Angular is a user-defined data transformation function that extends the framework’s capabilities. It allows developers to create reusable, specific data manipulation operations tailored to their application’s needs. Custom pipes are defined using the @Pipe decorator and can be used in Angular templates to format, filter, or transform data in various ways, such as custom sorting, filtering, or unique formatting requirements.
To create a custom pipe in Angular we have an In-built angular command.
ng generate pipe custom-pipe-name
We can also write that command in short form: 😄
ng g p custom-pipe-name
Instead of that “custom-pipe-name,” you can provide any desired name suitable to your custom-pipe file name.
Now after this custom file is created with by default standalone true flag. Its default
view looks like this:
You can modify this file according to your custom functions.
import { Pipe, PipeTransform } from '@angular/core';
@Pipe({
standalone: true,
name: 'customPipe'
})
export class CustomPipePipe implements PipeTransform {
transform(value: unknown, ...args: unknown[]): unknown {
return null;
}
}
To use this custom pipe throughout the system, you previously needed to import it into a module. However, with Angular 19 and standalone components, there are no modules. Instead, you can import the custom pipe only into the components where it is needed.
import { Component } from '@angular/core';
import { CommonModule } from '@angular/common';
import { customPipe} from './custom.pipe';
@Component({
selector: 'app-root',
standalone: true,
imports: [CommonModule, customPipe],
templateUrl: './app.component.html',
styleUrls: ['./app.component.scss']
})
export class AppComponent {
/** no-data */
}
Let see one Example of Custom Pipe:
Here creating custom pipe for searching data:
ng g p search
import {Pipe, PipeTransform} from '@angular/core';
/**
* search pipe for ngFor
*/
@Pipe({
standalone: true
name: 'search'
})
export class SearchPipe implements PipeTransform {
/**
* transform
*/
public transform(value, keys: string, term: string): Object {
if (!term) {
return value;
}
return (value || []).filter(item => keys.split(',').some(key =>
item.hasOwnProperty(key) && new RegExp(term, 'gi').test(item[key])));
}
}
In the Search Pipe file, you can write your own logic you want to apply to your template file. We have added logic for searching data in the ngFor loop in the template.
This will show only those records which includes “gi”.
One more thing to note about standalone pipes is that if a pipe is unused throughout the system, it is considered as “tree-shakable pipe.” This means unused pipes will not be included in the final build, thereby reducing the bundle size.
Frequently Asked Questions (FAQs)
1. Is it possible to chain impure pipes?
Yes, it is possible to chain impure pipe, but by doing so, it can degrade you application performance because impure pipe will execute frequently. It’s better to void chaining multiple impure pipes rather you can use services.
2. What is the use of JSON pipe apart from debugging object?
Apart from debugging, the JSON pipe in Angular can be used to display and format JSON data in templates, visualize complex objects, or export data in JSON format. It’s useful for showing raw data to users or preparing data for export. However, it’s best suited for development and debugging purposes, as using it on large datasets can impact performance due to the overhead of stringifying objects.
3. How to use a chaining pipe in a TypeScript le?
Let me quickly show you that. We will use UpperCase pipe and Slice pipe in typescript file to transform data.
public value: string = 'Hello, Angular!';
public finalTransformedValue: string = '';
constructor() {
// First, apply the uppercase pipe
let result = this.uppercasePipe.transform(this.value);
// Then, apply the slice pipe on the result
this.finalTransformedValue = this.slicePipe.transform(result, 0, 5);
}
4. Is it safer to use an impure pipe in a Template or in TypeScript?
For the best performance, it is generally advisable to use impure pipes in TypeScript for more complex or data-intensive operations, and reserve their use in templates for simpler, less performance-critical scenarios
That wraps up Angular pipes🎊! They simplify data transformations and enhance your templates. Explore, implement, and enjoy coding!!🙌